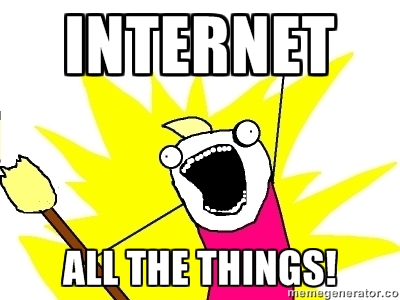
Like you I often spend my weekends writing REST APIs for fun. And like you I am often frustrated by the amount of server-side code I need to write just to expose some data to JavaScript. Boilerplate, boilerplate, boilerplate. Ugh! So this weekend, in between bouts of erratic and unsatisfying jetlag-sleep, I decided to try and simplify the task of exposing an API to JavaScript. Introducing “QuickModules for ASP.NET MVC”, available now as a NuGet package!
QuickModules
QuickModules provides you with a basic, modular framework for hanging API endpoints from. Internally using the Managed Extensibility Framework (MEF) to load modules, QuickModules gives you the ability to build encapsulated CRUD services without needing to write, or understand, the usual plumbing.
Consumer Experience
As a software developer, and a human (vaguely), I like an easy life, so I’m all about the consumer experience when publishing code or frameworks (see my JavaScript business-object CRUD framework at http://richstokoe.github.io).
In order to ease any concerns you may have I will run through all the steps required (there are very few) to get QuickModules into your MVC application.
Step 0: Open Visual Studio (Duh!)
Step 1: Create a new MVC project
Step 2: Choose an Empty, Basic, Internet, Intranet or SPA template (actually, any will do, but these make the most sense)
Step 3: Notice how barren your project is without QuickModules. 🙁 (I’ve chosen a Basic template here)
Step 4: Install QuickModules using NuGet: Right-click on the References node in your Solution Explorer tree and click on “Manage NuGet Packages”:
Step 5: Search for “QuickModules” and choose “Install”. (Don’t worry if the details and version numbers look a little different on your PC, this is a screenshot of an early work-in-progress package).
That’s it! Hit F5 to run your application. If you’ve chosen an Empty or Basic template you’ll probably get a 404 Not Found error. Try navigating to the sample “Nearby Restaurants” module by adding /QM/Get?ActivityType=NearbyRestaurants to your URL (QM being shorthand for QuickModules of course!).
You should see a list of food places from The Simpsons as a wonderful, unformatted, JSON object array, ready to be consumed by your JavaScript:
But Wait There’s More!
Built into the framework (and optionally supported by your modules) is paging, and filtering. All for the low, low price of $9.95. (Just kidding, it’s free). Use the LINQ-style Skip and Take arguments to control paging:
And use the Filter argument to narrow the result set down:
Code Dive! Geronimo!
Let’s have a look at the magic behind the scenes. First, you’ll notice that it’s pretty simple stuff. I’m a strong advocate of the KISS principle as it is usually the best way to keep things intuitively SOLID. In the same way that MEF is actually just a convention and a wrapper around .NET Reflection, QuickModules is actually just an abstraction layer to objects that implement an interface. It doesn’t really get more simple than that.
Let’s start at the QMController that has been added to your MVC project:
Nothing scary here. The JavaScript code calls /QM/Get/ and passes in the ActivityType and, optionally, Skip, Take and Filter arguments. For determinism, this is bound to a model called GetActivityRequest. “Activity” is the generic term for things that will be CRUD’d through QuickModules.
Note that we assign Request.QueryString to the ExtensionFields property of the GetActivityRequest. This allows your JavaScript to pass arguments to your module that aren’t natively supported by properties in the GetActivityRequest class. In short: you can change your JavaScript and module without having to worry about changing the QMController.
Next we pass the “request” object to ActivityManager.GetActivity(), which looks like this:
After a little defensive coding, we grab the current user as an IPrincipal (this lets you use whatever authentication mechanism you feel happy passing to modules) and then start looking for any module (or “activity provider”) that support the ActivityType given in the “request” parameter.
If we find one that supports the ActivityType, we check if it supports “Get”. If it does, we pass the module/provider the GetActivityRequest object.
The IActivityProvider interface requires us to provide an ActivityType property, which is used to identify the providers that support particular ActivityTypes (you can have multiple providers for an ActivityType. For example, you could have a provider that gets nearby restaurants from a web service, and another that gets them from a database. They will all be collated by the ActivityManager and spat back out to the consumer).
You can see here that I’m cheating slightly by building up the list of restaurants manually. Next, we apply the criteria from the GetActivityRequest to the List<Restaurant> and return it back to the ActivityManager.
If the ActivityManager can’t find any more providers that support “NearbyRestaurant”, this is the only data that will be returned.
Just a couple more things…
1. There’s no Update support yet.
2. A reference to System.ComponentModel.Composition will be added to your solution if it doesn’t already exist (this is to support the dynamic loading of modules).
So that’s it. Easy as pie. Go and get “QuickModules for ASP.NET MVC” now from NuGet. If you like it, give me a mention and don’t forget to rate it. If you don’t like it, don’t forget to tell me why!
Now go forth, hack, and have fun!
License
QuickModules comes with no warranty, express or implied. If anything breaks, it’s your fault, I’m not liable. Take backups before you install it. Feel free to update, modify, extend, steal, copy, delete (or any other operation you can think of) anything you want from the QuickModules source code.